Month: July 2023
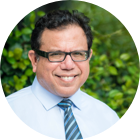
MMS • Giorgi Dalakishvili
Article originally posted on InfoQ. Visit InfoQ
JetBrains released an AI-powered version of ReSharper, its developer productivity extension for Microsoft Visual Studio. The new version, ReSharper 2023.2, is the first that will come with AI-powered development assistance.
The Early Access Program version includes a preview version of the AI assistant, a chat intended for programming questions. .NET developers using Visual Studio and ReSharper can use the AI assistant to troubleshoot or refactor existing code, generate code snippets or documentation and ask general questions about programming.
To access the AI-powered features users must log into JetBrains AI Services to enable the AI Assistant. When developers use AI features, some contextual information, such as file types, language, and framework used is shared with the provider of the large language model (LLM). The assistant does not have access to the source code unless developers include code fragments in their prompts. JetBrains also plans to support local and on-premises models so no data will be sent to the cloud-based LLM providers but the feature set will be rather limited.
The assistant takes into account the language and technologies of the projects and generates properly formatted code snippets. Users can copy and paste the generated code themselves or have the chatbot insert the code in the editor. The assistant also includes several prompts to get started.
Below is an example of what the assistant generates when prompted with “How do I find an element in a sorted array?” one of the prompts included in the assistant:
If developers use source code in the prompts, the source code will be sent to the LLM provider and the AI model will be able to generate, explain, or document code. For example, when asked, “How can I read the values from this query?” with the following code:
var connection = new SqliteConnection("DataSource=:memory:");
var command = connection.CreateCommand();
command.CommandText = "Select * from Item where value > ?";
command.Parameters.Add(4);
var reader = command.ExecuteReader();
The AI assistant generates code to consume the reader
and explains how it works:
To read the values from the query, you can use the SqliteDataReader
object that ExecuteReader() returns.
Here's an example of how you can do this:
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.WriteLine($"{reader.GetName(i)}: {reader.GetValue(i)}");
}
}
This code will loop through each row that the query returns. For each row, it will loop through each field and print the field’s name and value.
The assistant also spotted that in the initial code fragment, connection
, command
and reader
are not released, so it refactored the code to make sure they are properly cleaned up:
Please note that you should open the connection before executing
the command and close it after you're done.
Here's the complete code:
using (var connection = new SqliteConnection("DataSource=:memory:"))
{
connection.Open();
using (var command = connection.CreateCommand())
{
command.CommandText = "Select * from Item where value > ?";
command.Parameters.AddWithValue("value", 4);
using (var reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.WriteLine($"{reader.GetName(i)}: {reader.GetValue(i)}");
}
}
}
}
}
This code uses using statements to ensure that the SqliteConnection, SqliteCommand, and SqliteDataReader objects are correctly disposed of after use.
ReSharper also supports revisioning the prompts: if the original query needs revising or further information, developers can edit it instead of retyping the whole request. This will create a new chat with the assistant while preserving the original chat too. Furthermore, developers can organize the chats by creating, duplicating, renaming or deleting them.
The .NET community received the announcement with mixed feelings. Sébastien Lachance, a former Microsoft MVP said:
Usually, I don’t install beta version but when I saw AI Assistant in ReSharper, I could not resist. My first try was a success! My beloved tool ReSharper gets dramatically better.
Frans Bouma, creator and lead developer of a popular ORM for .NET, LLBLGen Pro, was disappointed:
Ugh, not you too… Come on! Invest in making the dev write *better* code instead of suggesting boiler plate goo that they won’t check if it’s wrong or not, resulting in crappy code that might succeed the tests but chances are these are generated by the goo generator too…
JetBrains AI assistant shares many commonalities with other AI-powered tools such as GitHub Copilot and Amazon CodeWhisperer but also adds unique features including chat history and accessing the assistant with ALT+Enter, the shortcut for everything.
The AI features are currently limited by the number of users and to the countries where OpenAI service is available. The AI service is free as part of the EAP program and the pricing information will be announced later. Apart from ReSharper, AI-powered assistant will be available in Rider as well as ReSharper for C++.
The release also introduced new quick-fixes and inspections for working with discard variables and inlay hints for LINQ queries. When debugging LINQ queries, developers will now see the intermediate output at each step of the query as inlay hints.
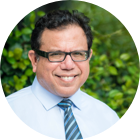
MMS • Ben Linders
Article originally posted on InfoQ. Visit InfoQ
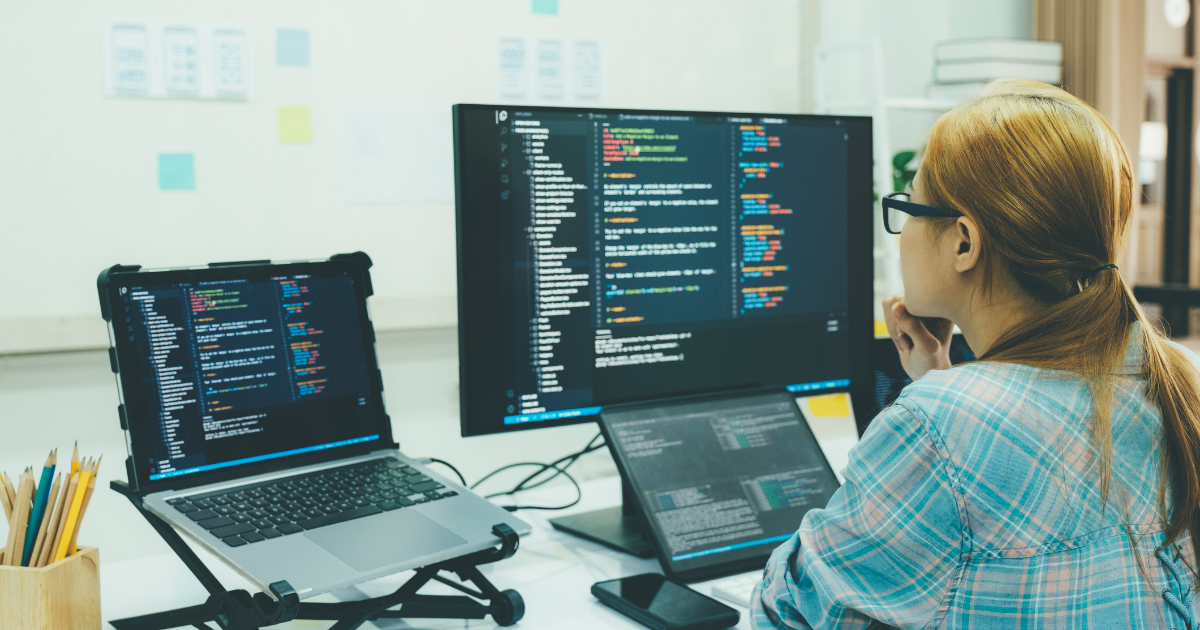
Property-based testing is an approach that involves specifying statements that should always be true, rather than relying on specific examples. It enables you to test functions across a large number of inputs with fewer tests.
Lucy Mair spoke about property-based testing at NDC Oslo 2023.
Property-based testing is a technique where one identifies properties – statements of the form “for all inputs that satisfy some precondition, some predicate holds” – and writes their tests using those, as Mair explained:
First, you write a function that specifies your property; it takes inputs that satisfy the precondition, then asserts the predicate holds. Next, you use a property-based testing framework which will generate many different inputs and run your function to check the predicate holds.
Mair presented examples using FsCheck and F#, but this technique can be used for any language, they said.
The most immediate benefit to writing property-based tests is being able to test functions across a large number of inputs without having to write a large number of tests, Mair said. Every run of a property-based test will use different inputs, which can give you confidence your code works in a general case. Mair mentioned that this is particularly valuable in finding edge cases for inputs you may never have thought to test.
Property-based tests work by generating and testing many cases so will take longer than traditional unit tests. The performance implications will vary based on your particular use case, Mair mentioned.
InfoQ interviewed Lucy Mair about property-based testing.
InfoQ: Can you give an example of a property?
Lucy Mair: Properties are related to functions, so let’s consider the case of a function concat(a, b) that concatenates two lists. As an example, concat([0, 1, 2], [3, 4]) = [0, 1, 2, 3, 4]
What statements can we write about c = concat(a, b) that are always true?
- The length of c will be a.length + b.length.
- All items within a will be present in c.
- All items within b will be present in c.
- Concatenating with an empty list returns the same list a = concat(a, []) = concat([], a).
InfoQ: How can we do property-based testing in front-end development?
Mair: Front-end development in JavaScript presents some unique challenges on the property-based testing front.
One issue is that JavaScript’s relatively lax runtime typing means generating an object of the correct shape for running the tests is non-trivial. TypeScript doesn’t help us here either; TypeScript types are compile-time only so cannot be used to create correctly shaped objects.
The second issue is that rendering components for front-end tests is slow, particularly if the test involves simulating user interactions. If your property-based tests include rendering they are likely to be unusably slow.
InfoQ: What are the potential downsides to property-based testing?
Mair: For property-based tests on pure functions, a traditional unit test may take e.g. 10 ms, and a property-based test may take e.g. 200 ms, so the performance impact is negligible. However, if your test is doing “slow” things, such as rendering a component, making API calls, or performing compute-intensive tasks then property-based tests may have too much of a performance overhead to use.
Introducing property-based tests to an existing code base may be a challenge. You are likely to have edge cases that may never arise during the running of the program and as such are never tested. An example of this is dividing by a number – zero is an invalid number in this case but you may know the number to never be non-zero due. This represents a specification that should be codified in tests but rarely is in practice.
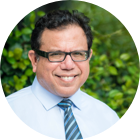
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
MongoDB, Inc. (NASDAQ:MDB – Get Free Report) saw some unusual options trading activity on Wednesday. Investors purchased 36,130 call options on the stock. This is an increase of 2,077% compared to the typical daily volume of 1,660 call options.
Analyst Upgrades and Downgrades
MDB has been the topic of several research analyst reports. Barclays increased their target price on MongoDB from $374.00 to $421.00 in a report on Monday, June 26th. Tigress Financial raised their price target on MongoDB from $365.00 to $490.00 in a research report on Wednesday, June 28th. VNET Group restated a “maintains” rating on shares of MongoDB in a research note on Monday, June 26th. 22nd Century Group reiterated a “maintains” rating on shares of MongoDB in a research note on Monday, June 26th. Finally, KeyCorp lifted their price objective on MongoDB from $229.00 to $264.00 and gave the company an “overweight” rating in a report on Thursday, April 20th. One research analyst has rated the stock with a sell rating, three have issued a hold rating and twenty have assigned a buy rating to the company. According to data from MarketBeat, MongoDB presently has an average rating of “Moderate Buy” and an average target price of $366.59.
MongoDB Stock Performance
MDB stock opened at $391.08 on Thursday. The stock’s 50 day simple moving average is $339.94 and its 200 day simple moving average is $257.00. The stock has a market capitalization of $27.60 billion, a PE ratio of -83.74 and a beta of 1.13. The company has a current ratio of 4.19, a quick ratio of 4.19 and a debt-to-equity ratio of 1.44. MongoDB has a 12-month low of $135.15 and a 12-month high of $418.70.
MongoDB (NASDAQ:MDB – Get Free Report) last issued its quarterly earnings data on Thursday, June 1st. The company reported $0.56 earnings per share for the quarter, beating analysts’ consensus estimates of $0.18 by $0.38. The firm had revenue of $368.28 million during the quarter, compared to analyst estimates of $347.77 million. MongoDB had a negative return on equity of 43.25% and a negative net margin of 23.58%. The business’s quarterly revenue was up 29.0% compared to the same quarter last year. During the same quarter last year, the business posted ($1.15) earnings per share. As a group, research analysts forecast that MongoDB will post -2.8 earnings per share for the current fiscal year.
Insider Buying and Selling at MongoDB
In other MongoDB news, CAO Thomas Bull sold 516 shares of the company’s stock in a transaction dated Monday, July 3rd. The shares were sold at an average price of $406.78, for a total value of $209,898.48. Following the transaction, the chief accounting officer now directly owns 17,190 shares in the company, valued at approximately $6,992,548.20. The transaction was disclosed in a legal filing with the SEC, which is available through the SEC website. In other news, CAO Thomas Bull sold 516 shares of the stock in a transaction that occurred on Monday, July 3rd. The stock was sold at an average price of $406.78, for a total transaction of $209,898.48. Following the completion of the transaction, the chief accounting officer now directly owns 17,190 shares in the company, valued at approximately $6,992,548.20. The transaction was disclosed in a document filed with the SEC, which is accessible through this link. Also, Director Dwight A. Merriman sold 2,000 shares of the business’s stock in a transaction on Wednesday, April 26th. The shares were sold at an average price of $240.00, for a total transaction of $480,000.00. Following the transaction, the director now directly owns 1,225,954 shares of the company’s stock, valued at approximately $294,228,960. The disclosure for this sale can be found here. In the last three months, insiders have sold 117,427 shares of company stock valued at $41,364,961. 4.80% of the stock is owned by company insiders.
Hedge Funds Weigh In On MongoDB
A number of institutional investors have recently modified their holdings of the stock. 1832 Asset Management L.P. grew its holdings in MongoDB by 3,283,771.0% in the fourth quarter. 1832 Asset Management L.P. now owns 1,018,000 shares of the company’s stock worth $200,383,000 after purchasing an additional 1,017,969 shares during the last quarter. Price T Rowe Associates Inc. MD lifted its position in MongoDB by 13.4% during the first quarter. Price T Rowe Associates Inc. MD now owns 7,593,996 shares of the company’s stock valued at $1,770,313,000 after purchasing an additional 897,911 shares during the period. Renaissance Technologies LLC lifted its position in MongoDB by 493.2% during the fourth quarter. Renaissance Technologies LLC now owns 918,200 shares of the company’s stock valued at $180,738,000 after purchasing an additional 763,400 shares during the period. Norges Bank acquired a new position in MongoDB during the fourth quarter valued at $147,735,000. Finally, Champlain Investment Partners LLC purchased a new position in MongoDB in the first quarter valued at $89,157,000. 89.22% of the stock is owned by hedge funds and other institutional investors.
MongoDB Company Profile
MongoDB, Inc provides general purpose database platform worldwide. The company offers MongoDB Atlas, a hosted multi-cloud database-as-a-service solution; MongoDB Enterprise Advanced, a commercial database server for enterprise customers to run in the cloud, on-premise, or in a hybrid environment; and Community Server, a free-to-download version of its database, which includes the functionality that developers need to get started with MongoDB.
Further Reading
This instant news alert was generated by narrative science technology and financial data from MarketBeat in order to provide readers with the fastest and most accurate reporting. This story was reviewed by MarketBeat’s editorial team prior to publication. Please send any questions or comments about this story to contact@marketbeat.com.
Before you consider MongoDB, you’ll want to hear this.
MarketBeat keeps track of Wall Street’s top-rated and best performing research analysts and the stocks they recommend to their clients on a daily basis. MarketBeat has identified the five stocks that top analysts are quietly whispering to their clients to buy now before the broader market catches on… and MongoDB wasn’t on the list.
While MongoDB currently has a “Moderate Buy” rating among analysts, top-rated analysts believe these five stocks are better buys.
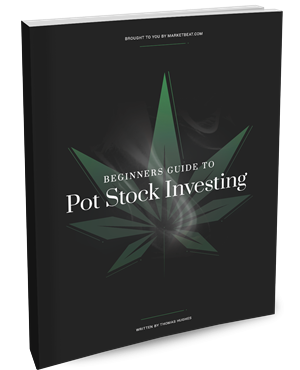
Click the link below and we’ll send you MarketBeat’s guide to pot stock investing and which pot companies show the most promise.
Article originally posted on mongodb google news. Visit mongodb google news
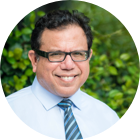
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
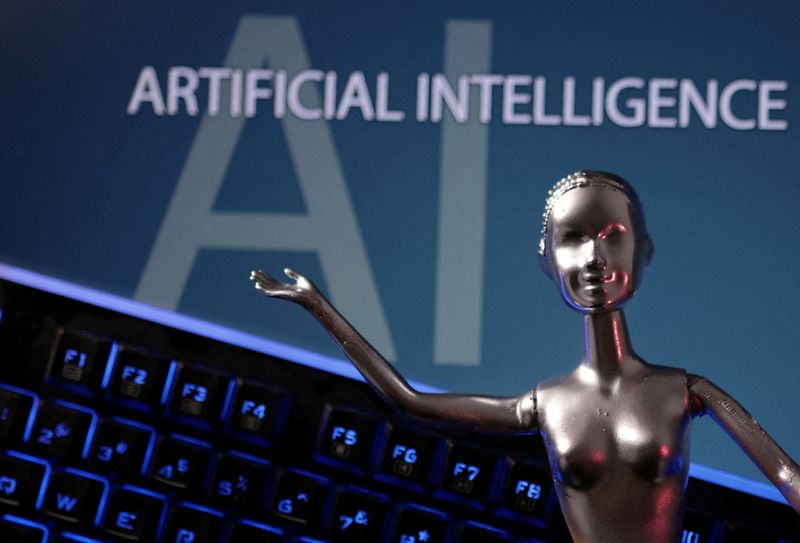
(Reuters) – AI startup Nomic has raised $17 million in a new funding round from investors led by Coatue, the companies told Reuters.
The investment valued New York-based Nomic AI, a team of four at the time, at $100 million, showing continued interest from VCs to bet on small teams building popular AI products. Contrary Capital and Betaworks Ventures also participated in the round.
Founded in 2022, Nomic has released two products to date, including an open-source AI model, GPT4ALL, which is free to download and could run on devices such as a laptop. It also has a tool called Atlas that allows users to visualize unstructured datasets used to build large language models (LLM).
Nomic said its goal is to boost the visibility of datasets in model training, and democratize access to AI models.
“We want to allow people to have access to the most powerful models that are specialized for the use cases they care about, and to build those systems themselves. They need to be able to understand what data goes into those systems,” said Andriy Mulyar, co-founder at Nomic AI, who plans to use the funding for hiring and product development.
Nomic said its products have been used by over 50,000 developers from companies including Hugging Face. It also has partnerships with MongoDB and Replit.
Open-source models are seen as alternatives to proprietary models developed by AI labs such as OpenAI and Google, as researchers around the world collaborate to develop models that could rival GPT-4.
“It gives you the platform for privacy with a local model, which will become more important as people have unique data that they want to interact with LLM,” said Sri Viswanath, partner at Coatue.
Coatue has made a series investment in open-source AI startups, including Stability AI, Hugging Face and Replit.
(Reporting by Krystal Hu in New York; Editing by Lincoln Feast.)
Article originally posted on mongodb google news. Visit mongodb google news
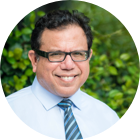
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
Introduction:
The New Report By 360 Research Reports Titled, ‘Global “Cloud-based Database Market” Size, Share, Price, Trends,Report and Forecast 2023-2029’, gives an in-depth analysis of the global Cloud-based Database market, assessing the market based on its segments like fraction, application, end-use, and major regions.The Cloud-based Database Market Report Contains 120 pages Including Full TOC, Tables and Figures, and Chart with In-depth Analysis Pre and Post COVID-19 Market Outbreak Impact Analysis and Situation by Region.
Who is the largest manufacturers of Cloud-based Database Market worldwide?
- Teradata
- MongoDB
- Alibaba
- Rackspace Hosting
- Microsoft
- Couchbase
- SAP
- Salesforce
- IBM
- Oracle
- Cassandra
- Tencent
- Amazon Web Services
Get a Sample PDF of report –https://www.360researchreports.com/enquiry/request-sample/21460458
Short Description About Cloud-based Database Market:
The Global Cloud-based Database market is anticipated to rise at a considerable rate during the forecast period, between 2022 and 2029. In 2021, the market is growing at a steady rate and with the rising adoption of strategies by key players, the market is expected to rise over the projected horizon.
The global Cloud-based Database market size was valued at USD 6188.5 million in 2021 and is expected to expand at a CAGR of 24.02Percent during the forecast period, reaching USD 22519.86 million by 2027.
A cloud database is a type of database service that is built, deployed and delivered through a cloud platform. It is primarily a cloud Platform as a Service (PaaS) delivery model that allows organizations, end users and their applications to store, manage and retrieve data from the cloud.
The report combines extensive quantitative analysis and exhaustive qualitative analysis, ranges from a macro overview of the total market size, industry chain, and market dynamics to micro details of segment markets by type, application and region, and, as a result, provides a holistic view of, as well as a deep insight into the Cloud-based Database market covering all its essential aspects.
For the competitive landscape, the report also introduces players in the industry from the perspective of the market share, concentration ratio, etc., and describes the leading companies in detail, with which the readers can get a better idea of their competitors and acquire an in-depth understanding of the competitive situation. Further, mergers and acquisitions, emerging market trends, the impact of COVID-19, and regional conflicts will all be considered.
In a nutshell, this report is a must-read for industry players, investors, researchers, consultants, business strategists, and all those who have any kind of stake or are planning to foray into the market in any manner.
Get a Sample Copy of the Cloud-based Database Report 2023
What are the factors driving the growth of the Cloud-based Database Market?
Growing demand for below applications around the world has had a direct impact on the growth of the Cloud-based Database
- Large Enterprises
- Small and Medium Business
What are the types of Cloud-based Database available in the Market?
Based on Product Types the Market is categorized into Below types that held the largest Cloud-based Database market share In 2022.
- NoSQL Database
- SQL Database
Which regions are leading the Cloud-based Database Market?
- North America (United States, Canada and Mexico)
- Europe (Germany, UK, France, Italy, Russia and Turkey etc.)
- Asia-Pacific (China, Japan, Korea, India, Australia, Indonesia, Thailand, Philippines, Malaysia and Vietnam)
- South America (Brazil, Argentina, Columbia etc.)
- Middle East and Africa (Saudi Arabia, UAE, Egypt, Nigeria and South Africa)
Inquire more and share questions if any before the purchase on this report at –https://www.360researchreports.com/enquiry/pre-order-enquiry/21460458
This Cloud-based Database Market Research/Analysis Report Contains Answers to your following Questions
- What are the global trends in the Cloud-based Database market? Would the market witness an increase or decline in the demand in the coming years?
- What is the estimated demand for different types of products in Cloud-based Database? What are the upcoming industry applications and trends for Cloud-based Database market?
- What Are Projections of Global Cloud-based Database Industry Considering Capacity, Production and Production Value? What Will Be the Estimation of Cost and Profit? What Will Be Market Share, Supply and Consumption? What about Import and Export?
- Where will the strategic developments take the industry in the mid to long-term?
- What are the factors contributing to the final price of Cloud-based Database? What are the raw materials used for Cloud-based Database manufacturing?
- How big is the opportunity for the Cloud-based Database market? How will the increasing adoption of Cloud-based Database for mining impact the growth rate of the overall market?
- How much is the global Cloud-based Database market worth? What was the value of the market In 2020?
- Who are the major players operating in the Cloud-based Database market? Which companies are the front runners?
- Which are the recent industry trends that can be implemented to generate additional revenue streams?
- What Should Be Entry Strategies, Countermeasures to Economic Impact, and Marketing Channels for Cloud-based Database Industry?
Cloud-based Database Market – Covid-19 Impact and Recovery Analysis:
We were monitoring the direct impact of covid-19 in this market, further to the indirect impact from different industries. This document analyzes the effect of the pandemic on the Cloud-based Database market from a international and nearby angle. The document outlines the marketplace size, marketplace traits, and market increase for Cloud-based Database industry, categorised with the aid of using kind, utility, and patron sector. Further, it provides a complete evaluation of additives concerned in marketplace improvement in advance than and after the covid-19 pandemic. Report moreover done a pestel evaluation within the business enterprise to study key influencers and boundaries to entry.
Our studies analysts will assist you to get custom designed info to your report, which may be changed in phrases of a particular region, utility or any statistical info. In addition, we’re constantly inclined to conform with the study, which triangulated together along with your very own statistics to make the marketplace studies extra complete for your perspective.
Final Report will add the analysis of the impact of Russia-Ukraine War and COVID-19 on this Cloud-based Database Industry.
TO KNOW HOW COVID-19 PANDEMIC AND RUSSIA UKRAINE WAR WILL IMPACT THIS MARKET – REQUEST SAMPLE
Detailed TOC of Global Cloud-based Database Market Research Report, 2023-2030
1 Market Overview
1.1 Product Overview and Scope of Cloud-based Database
1.2 Classification of Cloud-based Database by Type
1.2.1 Overview: Global Cloud-based Database Market Size by Type: 2017 Versus 2021 Versus 2030
1.2.2 Global Cloud-based Database Revenue Market Share by Type in 2021
1.3 Global Cloud-based Database Market by Application
1.3.1 Overview: Global Cloud-based Database Market Size by Application: 2017 Versus 2021 Versus 2030
1.4 Global Cloud-based Database Market Size and Forecast
1.5 Global Cloud-based Database Market Size and Forecast by Region
1.6 Market Drivers, Restraints and Trends
1.6.1 Cloud-based Database Market Drivers
1.6.2 Cloud-based Database Market Restraints
1.6.3 Cloud-based Database Trends Analysis
2 Company Profiles
2.1 Company
2.1.1 Company Details
2.1.2 Company Major Business
2.1.3 Company Cloud-based Database Product and Solutions
2.1.4 Company Cloud-based Database Revenue, Gross Margin and Market Share (2019, 2020, 2021 and 2023)
2.1.5 Company Recent Developments and Future Plans
3 Market Competition, by Players
3.1 Global Cloud-based Database Revenue and Share by Players (2019,2020,2021, and 2023)
3.2 Market Concentration Rate
3.2.1 Top3 Cloud-based Database Players Market Share in 2021
3.2.2 Top 10 Cloud-based Database Players Market Share in 2021
3.2.3 Market Competition Trend
3.3 Cloud-based Database Players Head Office, Products and Services Provided
3.4 Cloud-based Database Mergers and Acquisitions
3.5 Cloud-based Database New Entrants and Expansion Plans
4 Market Size Segment by Type
4.1 Global Cloud-based Database Revenue and Market Share by Type (2017-2023)
4.2 Global Cloud-based Database Market Forecast by Type (2023-2030)
5 Market Size Segment by Application
5.1 Global Cloud-based Database Revenue Market Share by Application (2017-2023)
5.2 Global Cloud-based Database Market Forecast by Application (2023-2030)
6 Regions by Country, by Type, and by Application
6.1 Cloud-based Database Revenue by Type (2017-2030)
6.2 Cloud-based Database Revenue by Application (2017-2030)
6.3 Cloud-based Database Market Size by Country
6.3.1 Cloud-based Database Revenue by Country (2017-2030)
6.3.2 United States Cloud-based Database Market Size and Forecast (2017-2030)
6.3.3 Canada Cloud-based Database Market Size and Forecast (2017-2030)
6.3.4 Mexico Cloud-based Database Market Size and Forecast (2017-2030)
7 Research Findings and Conclusion
8 Appendix
8.1 Methodology
8.2 Research Process and Data Source
8.3 Disclaimer
9 Research Methodology
10 Conclusion
Continued….
Purchase this report (Price 3250 USD for a single-user license) –https://www.360researchreports.com/purchase/21460458
About Us:
360 Research Reports is the credible source for gaining the market reports that will provide you with the lead your business needs. At 360 Research Reports, our objective is providing a platform for many top-notch market research firms worldwide to publish their research reports, as well as helping the decision makers in finding most suitable market research solutions under one roof. Our aim is to provide the best solution that matches the exact customer requirements. This drives us to provide you with custom or syndicated research reports.
Article originally posted on mongodb google news. Visit mongodb google news
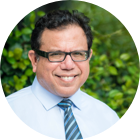
MMS • Steef-Jan Wiggers
Article originally posted on InfoQ. Visit InfoQ
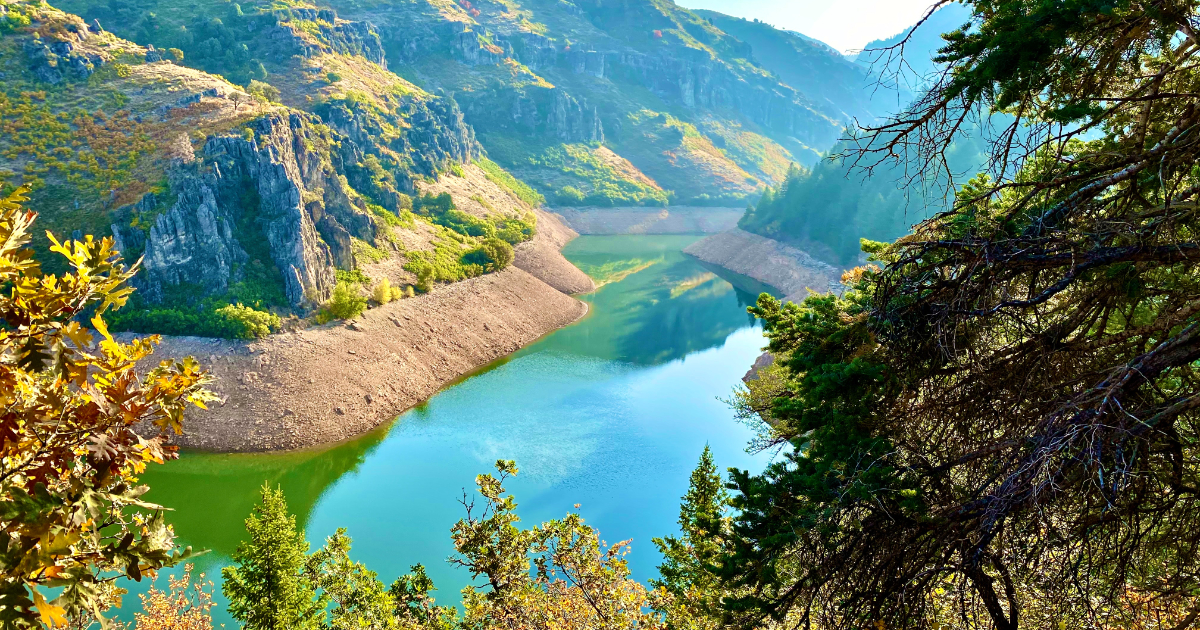
Recently AWS announced the preview release of the AWS .NET Distributed Cache Provider for DynamoDB. This library enables Amazon DynamoDB to be used as the storage for ASP.NET Core’s distributed cache framework.
The AWS .NET Distributed Cache Provider for DynamoDB is the successor to the AWS DynamoDB Session State Provider, offering compatibility with both .NET Framework and ASP.NET Core applications. While the session state provider library, released in 2012, is limited to .NET Framework applications, the new distributed cache provider also extends its functionality to support ASP.NET Core.
Alex Shovlin, software development engineer at AWS, explains in an AWS Developer Tools blog post the benefit of caching:
A cache can improve the performance of an application; an external cache allows the data to be shared across application servers and helps to avoid cache misses when the application is restarted or redeployed.
To use the library, developers can install the AWS.AspNetCore.DistributedCacheProvider package from NuGet.org. For instance, when they are building an application that requires sessions in a distributed webapp, .NET’s session state middleware looks for an implementation of IDistributedCache to store the session data. They can accomplish that by directing the session service to use the DynamoDB distributed cache implementation through dependency injection.
The company recommends configuring the cache provider with the following options for production applications:
- A Tablename, the name of the DynamoDB table that will store the cache data
- A PartitionKeyName, the name of the DynamoDB table’s partition key
- A TTLAttributeName, a DynamoDB’s Time To Live (TTL) feature, which removes cache items from the table
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddAWSDynamoDBDistributedCache(options =>
{
options.TableName = "session_cache_table";
options.PartitionKeyName = "id";
options.TTLAttributeName = "cache_ttl";
});
builder.Services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromSeconds(90);
options.Cookie.IsEssential = true;
});
var app = builder.Build();
...
In addition, Shovlin points out in the blog post that:
- The partition key must be of a type string without a sort key required for the table to prevent exceptions during cache operations
- Enable DynamoDB’s Time to Live feature for the table to ensure expired cached entries are automatically deleted
- Prefix the partition keys of cached items with a configurable value (default prefix is “dc:”) to avoid collisions and enable fine-grained access control using IAM policy conditions
- Additionally, consider using CreateTableIfNotExists for development or testing purposes, as it allows automatic table creation but may add latency to the first cache operation
Azure Cosmos DB, a comparable managed service like DynamoDB on Azure, can also serve as a cache for the session state. A Microsoft Caching Extension using Azure Cosmos DB package contains an implementation of IDistributedCache. This package can be leveraged in ASP.NET Core as a Session State Provider.
Alternatively, developers can use Amazon Relational Database Service (Amazon RDS) for SQL Server and Amazon ElastiCache for Redis for caching regarding ASP.NET Core applications. Both have an implementation of IDistributedCache: Microsoft.Extensions.Caching.SqlServer and Microsoft.Extensions.Caching.StackExchangeRedis.
The authors of a Microsoft Workloads on AWS blog post last year concluded:
AWS provides several managed services that can serve as a managed cache for your ASP.NET Core web applications. We examined how Amazon RDS for SQL Server and Amazon ElastiCache for Redis can be used as a distributed cache layer for your applications. They are managed services that are simple to set up and scale, with low operational overhead.
With AWS .NET Distributed Cache Provider for DynamoDB library, there is an additional now with Amazon DynamoDB.
The Microsoft documentation shows more details on using an IDistributedCache implementation to save session state data. In addition, the .NET on AWS page provides information on learning about building, deploying, and developing .NET applications on AWS.
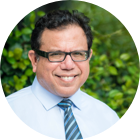
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
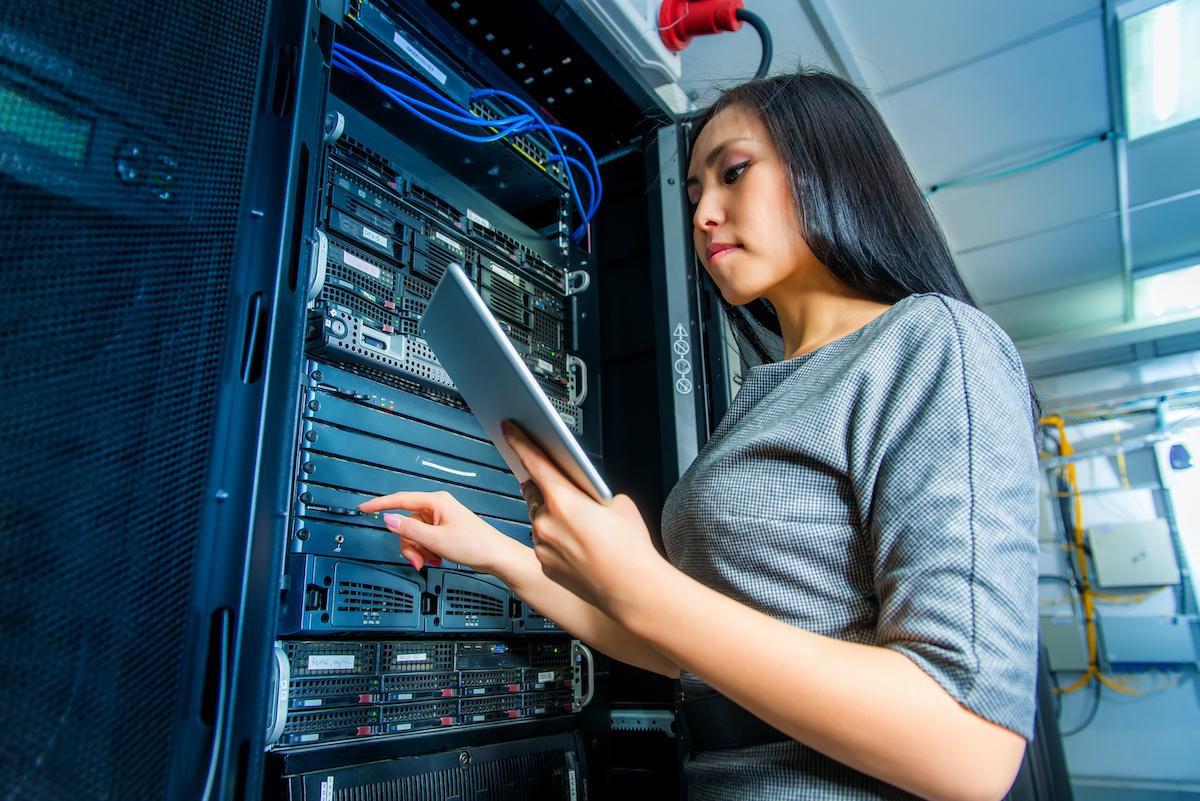
Database administrators have a tough job. They’re expected to create foundational, secure systems that support an entire organization’s data efforts—but unless something goes wrong with a database migration or the system is hit with unexpected downtime (to name just two problems these specialists are expected to solve), their efforts are often unrecognized by their colleagues.
Nonetheless, from a pure tech perspective, database administration is a rewarding career with many interesting problems to solve, along with enough dynamism to keep anyone happy long-term. To find out what skills truly make a great database administrator, we spoke to several experts with deep experience in the field.
What does a database administrator do day-to-day?
A database administrator leads the design, implementation, maintenance, and security implementation of a database. Database admins work closely with software developers, system administrators, and IT staff to ensure that databases are functioning properly, and the data within is accessible to users, not corrupted, and, most of all, secure from outside agencies.
What skills do database administrators need?
Database administrators must have a strong understanding of database theory and the technologies used to build and implement them. They also need to be able to work with a variety of database software products as required by their employer, and they need to be able to communicate effectively with other technical staff and with users of the database.
How much does a database administrator make?
According to the latest Dice Tech Salary Report, the average database administrator makes $107,828 per year, a year-over-year decline of 3.2 percent. Despite that decline, the job remains very much in demand; organizations everywhere need their databases spun up and maintained. Those database administrators with considerable experience can easily earn far more, especially if they have the specialized knowledge necessary for certain industries such as healthcare.
What platforms should database administrator master?
“There are several key ones to consider,” says Ivan Novak, Head of IT and Energy Casino. “First and foremost is Oracle, as it remains one of the leading database management systems in the industry. Its widespread use and features make it a valuable skill to possess. Another platform worth mastering is Microsoft SQL Server. With its popularity in many organizations, having expertise in SQL Server can open up numerous opportunities for database administrators.
Novak adds: “MySQL is an open-source database that is widely used for web applications. Gaining proficiency in MySQL can be advantageous as it provides flexibility and scalability. As NoSQL databases gain popularity, mastering MongoDB can also be beneficial. MongoDB’s document-oriented approach and ability to handle large amounts of data make it a valuable platform to understand. Lastly, PostgreSQL, known for its reliability, is another platform worth considering as it offers powerful features for database administration.”
Ruchi Agarwal, senior software engineer at Netflix and former software engineer at Apple, gives us her full list:
Oracle Database: Oracle is one of the most widely used enterprise-level database management systems (DBMS) and offers comprehensive tools and features for managing large-scale databases. Mastering Oracle Database administration, including tasks such as installation, configuration, performance tuning, backup and recovery, and security management, is highly valuable in many organizations.
Microsoft SQL Server: Microsoft SQL Server is another popular DBMS used in many organizations, particularly those using Microsoft technologies. Becoming proficient in SQL Server administration, including tasks such as installation, configuration, database design, query optimization, and high availability, can open up numerous job opportunities.
MySQL: MySQL is an open-source relational database management system commonly used in web applications and small to medium-sized enterprises. Understanding MySQL administration, including installation, configuration, performance tuning, and backup and recovery, can be beneficial, especially in environments that heavily rely on web-based applications.
PostgreSQL: PostgreSQL is a powerful open-source object-relational database system known for its robustness, extensibility, and support for advanced features. Mastering PostgreSQL administration, including tasks such as installation, configuration, performance optimization, replication, and security management, can provide you with in-demand skills for handling diverse database requirements.
MongoDB (optional): While the first four platforms mentioned are relational databases, it’s worth considering adding a NoSQL database like MongoDB to your skill set. MongoDB is a document-oriented database that offers flexibility, scalability, and high-performance for handling unstructured and semi-structured data. It is widely used in modern web and mobile applications. Having expertise in both SQL and NoSQL databases can be beneficial for a DBA’s career.
What technical skills do database administrators need?
Here is Agarwal’s list for technical skills, too:
- Database Management Systems (DBMS): DBAs should have a deep understanding of one or more DBMS platforms such as Oracle, Microsoft SQL Server, MySQL, PostgreSQL, or MongoDB. They should be proficient in tasks such as installation, configuration, maintenance, and troubleshooting of these systems.
- Database Design and Modeling: DBAs should possess strong skills in database design and data modeling. This includes creating efficient database schemas, defining relationships between tables, ensuring data integrity, and optimizing database structures for performance.
- SQL (Structured Query Language): SQL is the standard language used for interacting with relational databases. DBAs need to be well-versed in SQL to perform tasks like querying, updating, and managing databases. They should have expertise in writing complex SQL queries, optimizing SQL code, and understanding database optimization techniques.
- Performance Tuning and Optimization: DBAs should be proficient in monitoring and optimizing database performance. This includes identifying and resolving performance bottlenecks, optimizing SQL queries, configuring database parameters, implementing indexing strategies, and analyzing database statistics.
- Backup and Recovery: DBAs need to have a solid understanding of backup and recovery strategies to ensure the availability and integrity of data. This includes creating backup plans, scheduling backups, testing restore processes, and implementing disaster recovery plans.
- Security and Access Control: DBAs must have expertise in database security to protect sensitive data and ensure compliance with regulations. They should be skilled in implementing access control mechanisms, setting up user roles and permissions, encrypting data, and monitoring for security threats.
What soft skills are necessary for database administrators?
Vladislav Bilay, cloud DevOps engineer with Aquiva Labs, tells Dice: “In addition to technical expertise, database admins should know attention to detail is necessary for maintaining data accuracy and integrity. Additionally, time management and organizational skills help in prioritizing tasks and meeting deadlines. Communication skills are essential to interact with stakeholders, understand user requirements, and convey complex concepts in a clear manner. Problem-solving skills enable administrators to analyze issues and devise solutions efficiently.”
Novak agrees that communication is an essential soft skill for any database administrator: “Effective communication is essential for understanding user needs and collaborating with colleagues… As a one-person army, tackling complex problems and finding efficient solutions is crucial. The IT landscape is ever-evolving, so being adaptable and open to learning new technologies is essential. Attention to detail is also important as database administrators must ensure data integrity and security. Finally, collaborating with other IT professionals and working in a team environment is essential for larger projects.”
Can you get a job as a database administrator without a degree?
Given the demand for all kinds of tech specialists at the moment, recruiters and hiring managers are more willing than ever to hire database administrators and other tech pros who don’t necessarily have a formal degree—but you’ll need to demonstrate that you have the skills and experience to succeed in the role. No matter what your background, prepare for an in-depth technical interview that will require you to offer up solutions to hypothetical problems and show your knowledge of various tools and databases.
“While having a degree in a related field such as computer science or information technology can be advantageous, it is possible to get a job as a database administrator without a degree,” says Novak. “Employers often prioritize practical experience and relevant certifications. Building a strong portfolio of projects, acquiring certifications, and gaining hands-on experience through internships or entry-level positions can help compensate for the lack of a degree. Demonstrating technical competence, continuous learning, and a passion for the field can make you a competitive candidate even without a formal degree.”
“Yes, it is possible to get a job as a database administrator (DBA) without a degree,” Agarwal adds. “While many employers may prefer candidates with a degree in computer science, information technology, or a related field, the field of database administration also values practical experience, certifications, and relevant skills.” When trying to stand out in a crowded field of applicants, possessing certifications can help you stand out.
Article originally posted on mongodb google news. Visit mongodb google news
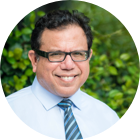
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
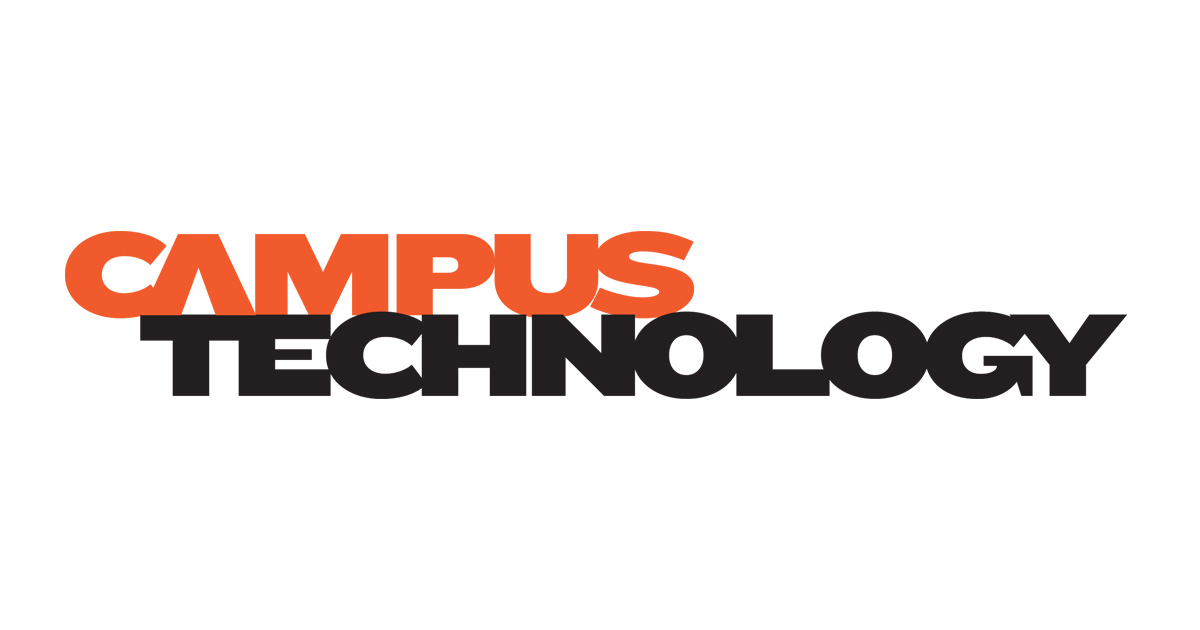
Software Development
MongoDB University Unveils Partnerships with Underrepresented Groups, LinkedIn Learning, Coursera
MongoDB University has announced new partnerships and initiatives to help close the widening software-development skills gap, according to a news release.
MongoDB has established distribution partnerships with Coursera and LinkedIn Learning to make the university’s software development courses more widely accessible, the organization said.
New partnerships with Women Who Code, MyTechDev, and Lesbians Who Tech & Allies will result in free certification for 700 students seeking careers as software developers, part of MongoDB’s efforts to “ensure that people from traditionally underrepresented groups have an opportunity to gain skills with MongoDB Atlas.”
Additionally, the MongoDB for Academia program now offers new benefits for educators such as free MongoDB Atlas credits and certifications, according to the announcement.
Since the relaunch of MongoDB University last November, more than 50,000 developers have accessed its free courses, the institution said, and more than 600 have earned certification as MongoDB software professionals.
Learn more at mongodb.com.
About the Author
Kristal Kuykendall is editor, 1105 Media Education Group. She can
be reached at [email protected].
Article originally posted on mongodb google news. Visit mongodb google news
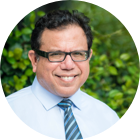
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
MongoDB, Inc. (NASDAQ:MDB – Get Free Report) was the target of some unusual options trading activity on Wednesday. Traders acquired 23,831 put options on the company. This is an increase of approximately 2,157% compared to the average volume of 1,056 put options.
MongoDB Price Performance
Shares of NASDAQ:MDB opened at $391.08 on Thursday. MongoDB has a fifty-two week low of $135.15 and a fifty-two week high of $418.70. The firm has a market cap of $27.60 billion, a price-to-earnings ratio of -83.74 and a beta of 1.13. The company has a debt-to-equity ratio of 1.44, a quick ratio of 4.19 and a current ratio of 4.19. The business has a 50-day simple moving average of $339.94 and a two-hundred day simple moving average of $257.00.
MongoDB (NASDAQ:MDB – Get Free Report) last released its quarterly earnings results on Thursday, June 1st. The company reported $0.56 EPS for the quarter, beating the consensus estimate of $0.18 by $0.38. The firm had revenue of $368.28 million during the quarter, compared to analyst estimates of $347.77 million. MongoDB had a negative net margin of 23.58% and a negative return on equity of 43.25%. The business’s revenue for the quarter was up 29.0% compared to the same quarter last year. During the same quarter in the prior year, the firm earned ($1.15) EPS. On average, analysts expect that MongoDB will post -2.8 earnings per share for the current year.
Insider Activity at MongoDB
In other MongoDB news, Director Dwight A. Merriman sold 606 shares of the business’s stock in a transaction dated Monday, July 10th. The shares were sold at an average price of $382.41, for a total value of $231,740.46. Following the transaction, the director now directly owns 1,214,159 shares in the company, valued at $464,306,543.19. The transaction was disclosed in a document filed with the SEC, which is available through this link. In related news, Director Dwight A. Merriman sold 2,000 shares of the stock in a transaction on Thursday, May 4th. The shares were sold at an average price of $240.00, for a total transaction of $480,000.00. Following the completion of the transaction, the director now owns 1,223,954 shares in the company, valued at $293,748,960. The sale was disclosed in a filing with the Securities & Exchange Commission, which is accessible through the SEC website. Also, Director Dwight A. Merriman sold 606 shares of the firm’s stock in a transaction dated Monday, July 10th. The stock was sold at an average price of $382.41, for a total transaction of $231,740.46. Following the sale, the director now owns 1,214,159 shares of the company’s stock, valued at approximately $464,306,543.19. The disclosure for this sale can be found here. Insiders have sold a total of 117,427 shares of company stock valued at $41,364,961 over the last quarter. 4.80% of the stock is owned by company insiders.
Institutional Inflows and Outflows
A number of hedge funds and other institutional investors have recently modified their holdings of the company. 1832 Asset Management L.P. increased its stake in MongoDB by 3,283,771.0% in the fourth quarter. 1832 Asset Management L.P. now owns 1,018,000 shares of the company’s stock worth $200,383,000 after purchasing an additional 1,017,969 shares during the period. Price T Rowe Associates Inc. MD raised its stake in shares of MongoDB by 13.4% in the first quarter. Price T Rowe Associates Inc. MD now owns 7,593,996 shares of the company’s stock worth $1,770,313,000 after purchasing an additional 897,911 shares during the last quarter. Renaissance Technologies LLC raised its position in MongoDB by 493.2% during the fourth quarter. Renaissance Technologies LLC now owns 918,200 shares of the company’s stock valued at $180,738,000 after acquiring an additional 763,400 shares in the last quarter. Norges Bank purchased a new position in MongoDB during the fourth quarter valued at approximately $147,735,000. Finally, Champlain Investment Partners LLC purchased a new position in MongoDB during the first quarter valued at approximately $89,157,000. 89.22% of the stock is currently owned by institutional investors.
Analyst Upgrades and Downgrades
Several brokerages recently issued reports on MDB. Royal Bank of Canada lifted their price target on shares of MongoDB from $400.00 to $445.00 in a report on Friday, June 23rd. 58.com restated a “maintains” rating on shares of MongoDB in a research report on Monday, June 26th. Robert W. Baird lifted their price objective on shares of MongoDB from $390.00 to $430.00 in a research note on Friday, June 23rd. Tigress Financial lifted their price objective on shares of MongoDB from $365.00 to $490.00 in a research note on Wednesday, June 28th. Finally, Oppenheimer boosted their price target on shares of MongoDB from $270.00 to $430.00 in a research note on Friday, June 2nd. One research analyst has rated the stock with a sell rating, three have issued a hold rating and twenty have assigned a buy rating to the company’s stock. According to MarketBeat, the stock has a consensus rating of “Moderate Buy” and a consensus price target of $366.59.
About MongoDB
MongoDB, Inc provides general purpose database platform worldwide. The company offers MongoDB Atlas, a hosted multi-cloud database-as-a-service solution; MongoDB Enterprise Advanced, a commercial database server for enterprise customers to run in the cloud, on-premise, or in a hybrid environment; and Community Server, a free-to-download version of its database, which includes the functionality that developers need to get started with MongoDB.
Featured Stories
Receive News & Ratings for MongoDB Daily – Enter your email address below to receive a concise daily summary of the latest news and analysts’ ratings for MongoDB and related companies with MarketBeat.com’s FREE daily email newsletter.
Article originally posted on mongodb google news. Visit mongodb google news
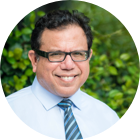
MMS • RSS
Posted on mongodb google news. Visit mongodb google news
MongoDB, Inc. (NASDAQ:MDB – Get Free Report) saw unusually large options trading on Wednesday. Stock traders bought 36,130 call options on the company. This represents an increase of 2,077% compared to the average volume of 1,660 call options.
Insider Transactions at MongoDB
In related news, Director Dwight A. Merriman sold 606 shares of the business’s stock in a transaction dated Monday, July 10th. The stock was sold at an average price of $382.41, for a total value of $231,740.46. Following the sale, the director now directly owns 1,214,159 shares in the company, valued at approximately $464,306,543.19. The sale was disclosed in a document filed with the Securities & Exchange Commission, which is available at this hyperlink. In other news, Director Dwight A. Merriman sold 606 shares of the company’s stock in a transaction dated Monday, July 10th. The stock was sold at an average price of $382.41, for a total transaction of $231,740.46. Following the sale, the director now directly owns 1,214,159 shares in the company, valued at $464,306,543.19. The sale was disclosed in a document filed with the SEC, which is available at this link. Also, CRO Cedric Pech sold 2,738 shares of the business’s stock in a transaction dated Wednesday, July 5th. The stock was sold at an average price of $406.18, for a total value of $1,112,120.84. Following the transaction, the executive now directly owns 34,418 shares of the company’s stock, valued at approximately $13,979,903.24. The disclosure for this sale can be found here. Insiders have sold 117,427 shares of company stock worth $41,364,961 in the last ninety days. Company insiders own 4.80% of the company’s stock.
Institutional Trading of MongoDB
A number of institutional investors have recently bought and sold shares of MDB. Bessemer Group Inc. purchased a new stake in MongoDB during the 4th quarter valued at approximately $29,000. BI Asset Management Fondsmaeglerselskab A S acquired a new stake in shares of MongoDB in the 4th quarter worth approximately $30,000. Global Retirement Partners LLC grew its holdings in shares of MongoDB by 346.7% in the 1st quarter. Global Retirement Partners LLC now owns 134 shares of the company’s stock worth $30,000 after acquiring an additional 104 shares during the period. Lindbrook Capital LLC grew its holdings in shares of MongoDB by 350.0% in the 4th quarter. Lindbrook Capital LLC now owns 171 shares of the company’s stock worth $34,000 after acquiring an additional 133 shares during the period. Finally, Y.D. More Investments Ltd acquired a new stake in shares of MongoDB in the 4th quarter worth approximately $36,000. 89.22% of the stock is owned by hedge funds and other institutional investors.
MongoDB Trading Down 0.8 %
MDB opened at $391.08 on Thursday. MongoDB has a 12 month low of $135.15 and a 12 month high of $418.70. The stock has a market capitalization of $27.60 billion, a price-to-earnings ratio of -83.74 and a beta of 1.13. The company has a debt-to-equity ratio of 1.44, a current ratio of 4.19 and a quick ratio of 4.19. The business has a fifty day moving average price of $339.94 and a 200 day moving average price of $257.00.
MongoDB (NASDAQ:MDB – Get Free Report) last announced its quarterly earnings results on Thursday, June 1st. The company reported $0.56 earnings per share (EPS) for the quarter, topping analysts’ consensus estimates of $0.18 by $0.38. The company had revenue of $368.28 million during the quarter, compared to the consensus estimate of $347.77 million. MongoDB had a negative return on equity of 43.25% and a negative net margin of 23.58%. MongoDB’s revenue for the quarter was up 29.0% on a year-over-year basis. During the same period in the previous year, the business earned ($1.15) EPS. Sell-side analysts expect that MongoDB will post -2.8 earnings per share for the current fiscal year.
Wall Street Analysts Forecast Growth
Several analysts have weighed in on the company. KeyCorp boosted their price objective on MongoDB from $229.00 to $264.00 and gave the company an “overweight” rating in a research report on Thursday, April 20th. William Blair reaffirmed an “outperform” rating on shares of MongoDB in a research report on Friday, June 2nd. Tigress Financial upped their price objective on MongoDB from $365.00 to $490.00 in a research report on Wednesday, June 28th. Capital One Financial started coverage on MongoDB in a research note on Monday, June 26th. They set an “equal weight” rating and a $396.00 price objective for the company. Finally, Citigroup raised their price objective on MongoDB from $363.00 to $430.00 in a report on Friday, June 2nd. One investment analyst has rated the stock with a sell rating, three have given a hold rating and twenty have issued a buy rating to the company. According to data from MarketBeat, MongoDB presently has an average rating of “Moderate Buy” and a consensus price target of $366.59.
MongoDB Company Profile
MongoDB, Inc provides general purpose database platform worldwide. The company offers MongoDB Atlas, a hosted multi-cloud database-as-a-service solution; MongoDB Enterprise Advanced, a commercial database server for enterprise customers to run in the cloud, on-premise, or in a hybrid environment; and Community Server, a free-to-download version of its database, which includes the functionality that developers need to get started with MongoDB.
Further Reading
Receive News & Ratings for MongoDB Daily – Enter your email address below to receive a concise daily summary of the latest news and analysts’ ratings for MongoDB and related companies with MarketBeat.com’s FREE daily email newsletter.
Article originally posted on mongodb google news. Visit mongodb google news